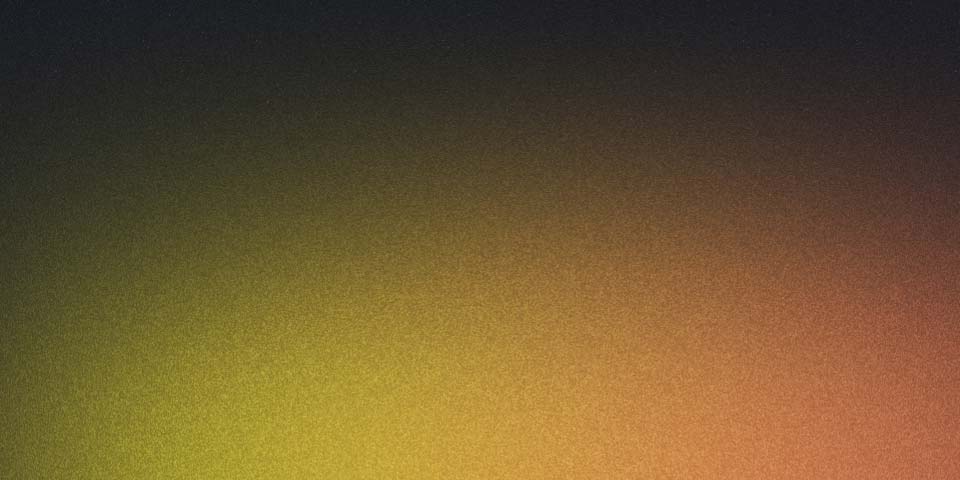
Follow account on Twitter/X using Playwright
We start with an authenticated account. If you’d like to know how to authenticate on Twitter/X using Playwright, read this post.
Much of the code is similar to what we did before. So let’s go straight to it
await goHome(page);
// Open a tweet
const showMore = await page.$("span:has-text('Show more')");
await showMore.click();
await page.waitForSelector("span:has-text('Post')");
// Go to the author's profile
const tweetUrl = page.url(); // https://x.com/username/status/2342345
const username = tweetUrl.split("/")[3];
await page.goto(`https://x.com/${username}`);
// Open its "following" list
await page.click("a[href*='/following']");
// follow the first 3 users
const followButtons = await page
.locator("//span[contains(text(), 'Follow')]")
.all();
for (let i = 0; i < 3; i++) {
await followButtons[i].click();
}
The source code is a bit different. It has intentional wait-times. My intention is to make the behavior less robotic and more human by introducing delays.
I’m still learning Playwright. While doing this, I learned how to get all elements using a locator.
Before, I only knew how to click on an element. This time, I didn’t want to click on all “Follow” buttons. So, I listed all
elements and then clicked on some of them.
Getting all elements is considered a rare case by Playwright, haha.
The code
Find the code here and a video demo below. Thanks for reading!